Overview
Important
Pynapse underwent a significant revision in v96. This documentation is for v96 and above. For Pynapse v95 documentation, see Pynapse Manual for v95 Synapse.
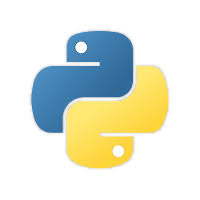
Pynapse is a gizmo for tightly integrating Python coding into your Synapse experiment.
Many users write external code in Python (or MATLAB) that runs alongside their Synapse experiments. These programs are used for overall experiment control, stimulus delivery, behavioral control, and online analysis - things that are either novel paradigms that don't exist in the current gizmo set or can't easily be programmed to run directly on the real-time hardware.
There are several challenges faced by these users and Pynapse is designed to address these issues with an intuitive and powerful interface.
Benefits of Pynapse
Pynapse is more than a great embedded Python editor within Synapse. You get:
- Great Python editor with all the bells and whistles (highlighting, code completion, and more)
- Easy-to-learn, structured programming framework
- Fully automatic Synapse synchronization. Your Python code is saved and version controlled with your experiment
- Powerful hardware (iCon or RZ I/O) and software seamlessly integrated
- Runtime live code monitoring
- Automatic code flowcharting
- Built-in trial and session controls
- Track experiment progress and plot results
Pynapse Gizmo
![]() |
Pynapse Gizmo Block Diagram |
All of this is built into the Pynapse gizmo. Use the Python installation provided in Synapse (or bring in your own) and drop the Pynapse gizmo into your Synapse experiment.
The circuit that runs on the real-time hardware has all the features that anyone writing custom Python code to interact with the hardware. Pynapse runs an optimized polling loop that synchronizes Python to Synapse, faster than existing methods. The State Machine architecture in Pynapse yields tight programs that are easy to read and easy to debug.
Event Loop
A tight polling loop is continuously running and monitoring hardware events defined in the experiment.
- Hardware event is detected by the Pynapse event loop.
- Call is made into Python to execute a method written by the user
- Call is logged and timestamped
- Events are sent back to the hardware
All of this happens in milliseconds.
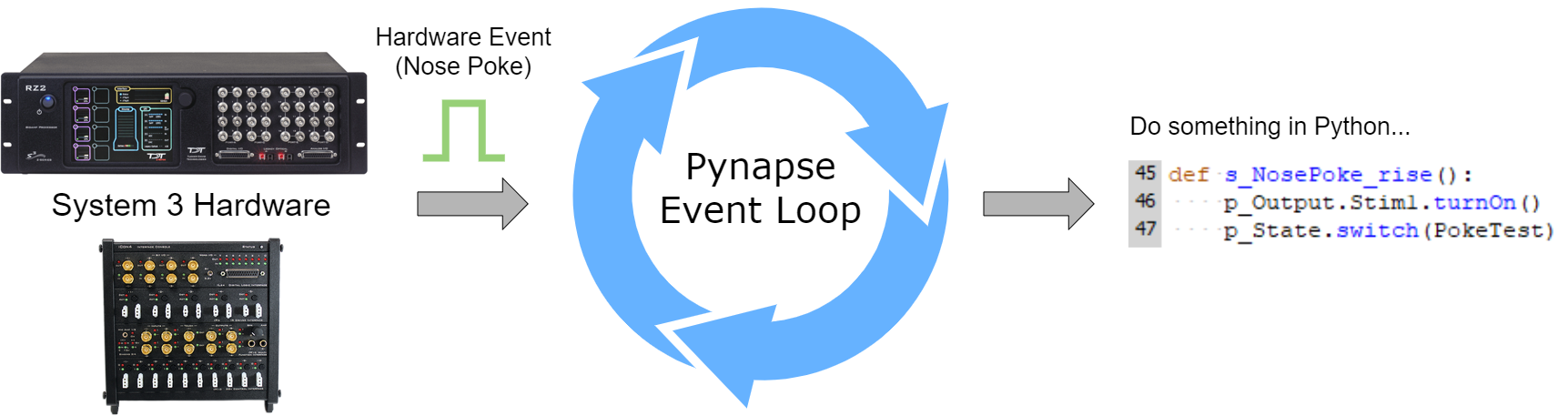
Python State Machine
The Python code can be organized into 'States'. Pynapse keeps track of which state it is in, and hardware events will only trigger Python calls defined within that state. State changes are also controlled by the Python code, and automatically timestamped and stored with the rest of your data for easy analysis.
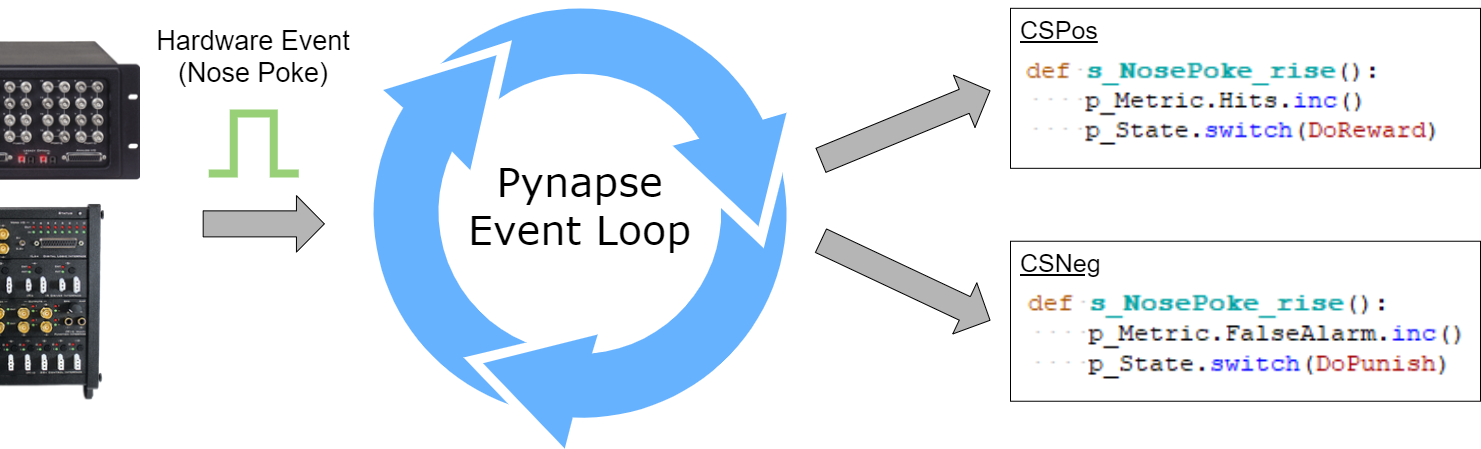
Session Manager
State changes make up trials. Trials can be organized into blocks, and sessions.
Define metrics that are logged and plotted at any of these intervals - per trial, per block, per
session, or any time they change. Simply set up the number of trials and blocks you want
to run, call startTrial
inside the Python state machine to initiate a trial, and the
rest is taken care of automatically.
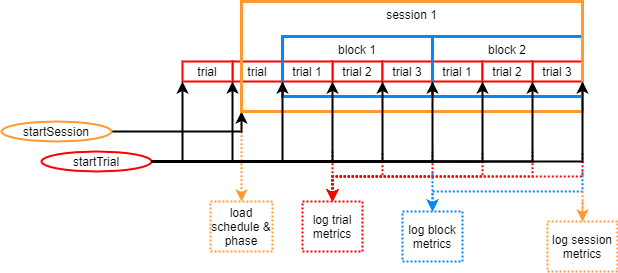
Take it another step further by organizing groups of experiment settings into Phases, then feed the session manager a schedule of the how many trials/block for each phase. For more open-ended experiments that don't have a fixed number of trials / blocks, you can control the entire trial/block/session flow manually from your Python script. See Sessions for more information.
iCon Integration
Pynapse runs in two different I/O modes. You can use the gizmo inputs and outputs to connect to RZ input/output links, or you can integrate an iCon module directly into Pynapse. Configure all iCon inputs and outputs in Pynapse, and access them in your Python code.
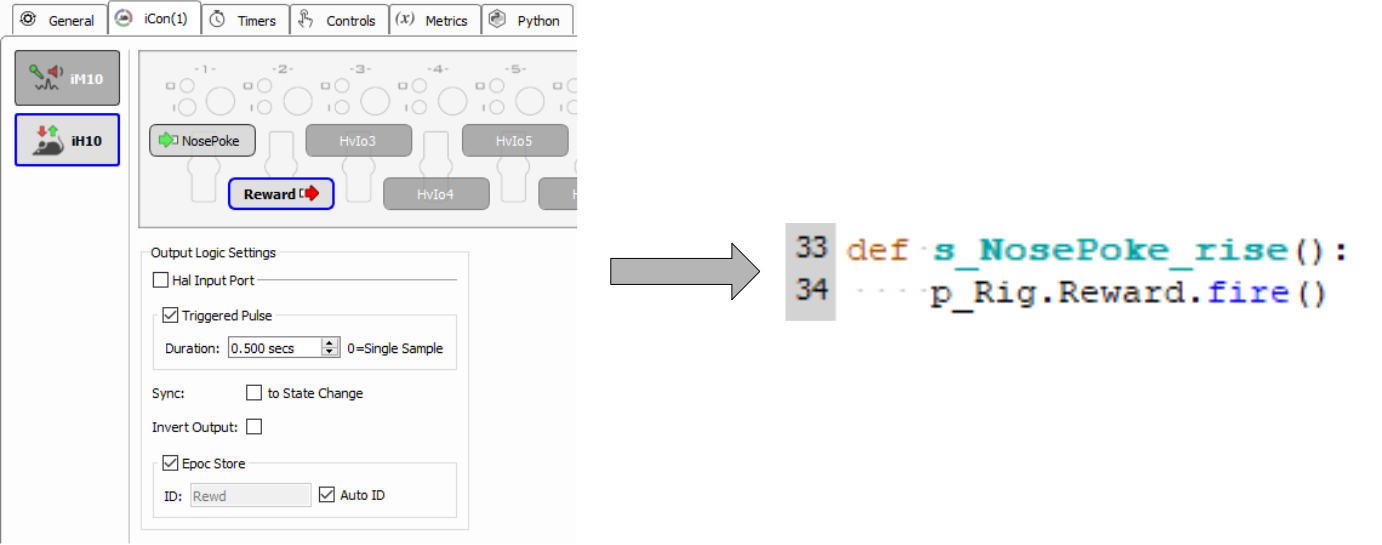
See iCon Inputs and iCon Outputs for more information.
Main Assets of Pynapse Gizmo
Pynapse has the following fundamental asset classes built into it that are accessible in the Python code.
Common Applications
Program Control
- Start/stop Synapse or other programs based on conditional triggers
- Run Synapse for a set duration
Behavioral Control
- Implement complex behavioral paradigms over trials that control:
- Cues
- Waiting periods
- Input decisions
- Reward output
- and more
Signal Analysis and Display
- Collect signals in a triggered buffer or through the API
- Perform calculations, such as:
- Presentation averaging, spike counting, or FFT
- Plot results using Python plotting libraries (such as Matplotlib)
Stimulus Presentation
- Generate simple or complex stimuli to present during triggered conditions using Pynapse output control or built-in buffers