Sessions
Session Mode Controls
Session Mode Controls allow you to schedule the number of trials and blocks to run. It triggers the trial, block, and session Metrics to save, display, and plot. It also includes a Scheduler which can automatically present different phases of the experiment with minimal user interaction.
Enable Session Controls in the Controls tab.
![]() |
Session Control Options |
The Session Mode Controls add a run-time interface to start, stop, pause, resume the session. Scheduler controls also appear at run-time. A session counter, block counter, and trial counter are also on the interface.
![]() |
Session Runtime Controls |
See Slot Methods for Responding to Session Changes for an example of how these might be used in your Python code.
Flow Control
The three Flow Control methods are described below. The diagrams show the session flow based on when the python Trial Control methods (colored ovals) are called. The tables show when the Status information methods return true (black in the timeline).
Automatic
In Automatic mode, the session starts immediately when you click the 'Start' button.
You only need to call p_Session.startTrial()
in your Python code during an active
session and the scheduler will automatically increment the trial and block counters for
you, based on the number of trials that have already occurred.
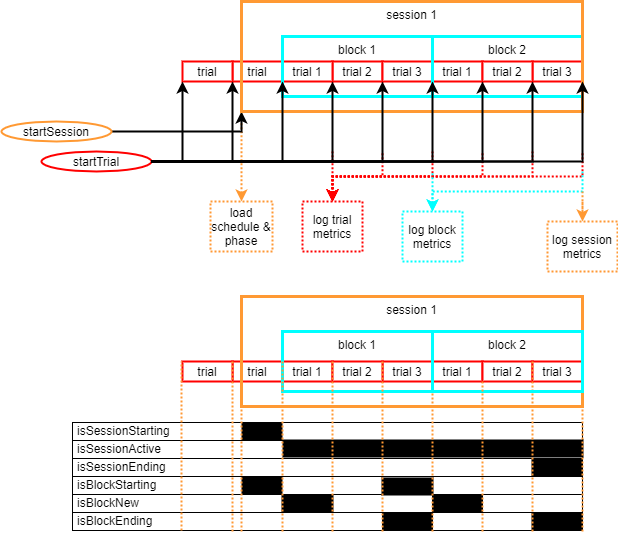
Automatic with SyncDelay
In Automatic with SyncDelay mode, the phase is loaded when the current trial completes
and the session starts after one full trial has finished after that.
You only need to call p_Session.startTrial()
in your Python code during an active
session and the scheduler will automatically increment the trial and block counters for
you, based on the number of trials that have already occurred.
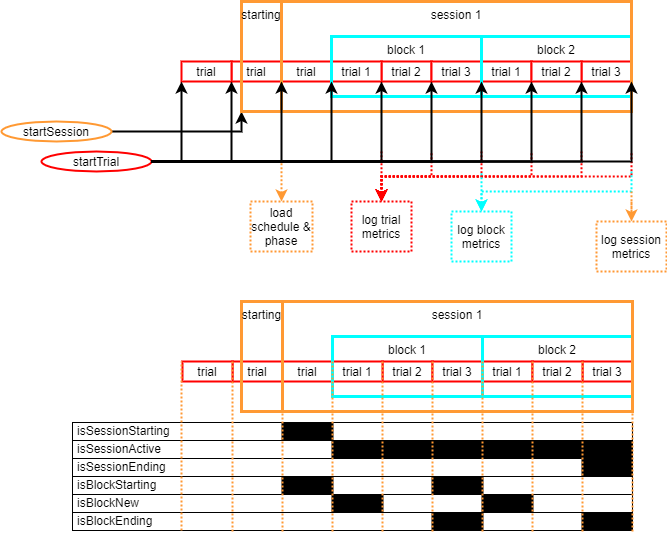
Manual
In Manual mode, you manually call p_Session.startBlock()
and
p_Session.startTrial()
in the Python code during an active session to advance the
trial and block counters. You can use the mode controls at the top
of the runtime interface or call p_Session.startSession()
and p_Session.stopSession()
in the Python code.
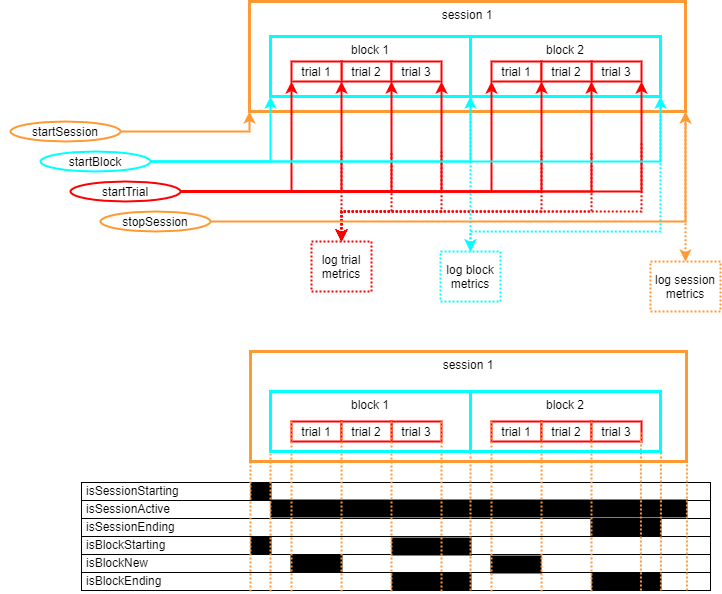
You can also optionally call p_Session.endTrial()
to add a gap between trials.
This call ends the trial and logs the trial metrics before starting the next trial.
You can use this time to make a decision before the next trial begin.
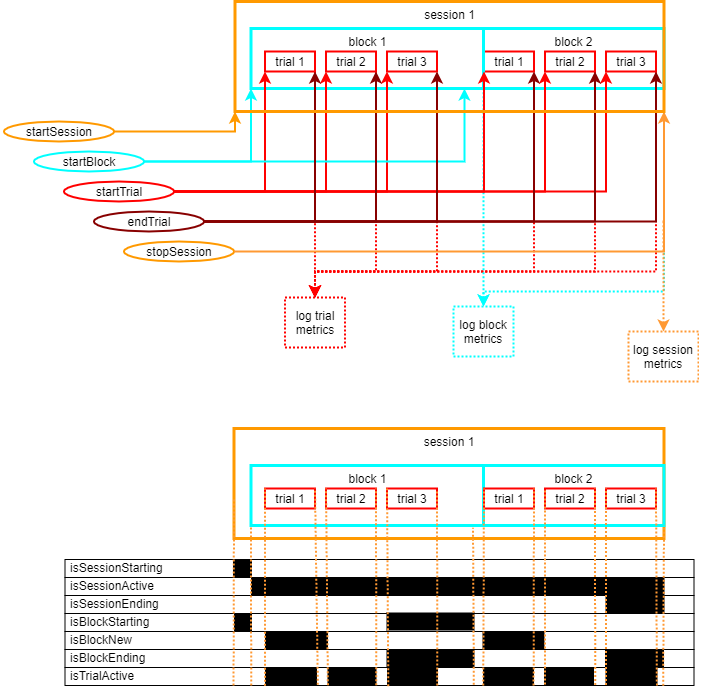
Note
isBlockStarting and isBlockEnding turn on when the trial count is reached.
isSessionEnding turns on when the block count and trial count are reached.
Scheduler
If you are using one of the 'Automatic' flow control methods and are using Phase Presets, then a run-time Scheduler manages the experiment flow. It is programmed with a list of phases and the number of trials and blocks to run for each phase.
![]() |
Session Scheduler Controls |
Enter the Phase ID (e.g. P1, P2) into the input boxes to set the scheduler. When the specified number of blocks and trials for the current phase has finished, the scheduler will automatically advance to the next phase in the list.
![]() |
Session Scheduler Phase List |
Enter the Phase ID, number of trials, and number of blocks as a comma-separated list to override the default trial and block count.
![]() |
Session Scheduler Trial and Block Specification |
Click the arrow button to end the current phase and start the next phase immediately on the next trial. The arrow button will turn red. Click it again before the next trial starts to undo this operation.
![]() |
Session Scheduler Override |
Slot Methods for Responding to Session Changes
These control slot methods capture status information about the session. They are available as method definitions inside Pynapse states. Write a method with this name to react to the corresponding event.
Important
The s_Session
methods are only available if Session Mode Controls is enabled
in the Controls tab.
Example
Switch to a starting state when user clicks the 'Start' button on the Pynapse tab at runtime.
class Always: # StateID = 0
def s_Session_start():
p_Metric.count.write(0)
p_State.switch(PreTrial)
def s_Session_pause():
p_Metric.count.write(0)
p_State.switch(Resting)
def s_Session_resume():
p_State.switch(PreTrial)
def s_Session_stop():
print(p_Metric.ntrials.read(), 'trials completed')
p_State.switch(EndTrials)
Methods
All state methods have the form p_Session.{METHOD}
. Type p_
in the Pynapse Code Editor
and let the code completion do the work for you.
Session Control
startSession
Mimics the behavior of clicking the 'Start' button.
pauseSession
p_Session.pauseSession()
Mimics the behavior of clicking the 'Pause' button.
resumeSession
p_Session.resumeSession()
Mimics the behavior of clicking the 'Resume' button.
stopSession
p_Session.stopSession()
Mimics the behavior of clicking the 'Stop' button.
disabManSessionControl
p_Session.disabManSessionControl()
Disables the Start/Stop/Pause/Resume buttons on the run-time interface.
enabManSessionControl
p_Session.enabManSessionControl()
Enables the Start/Stop/Pause/Resume buttons on the run-time interface.
Trial Control
setTrialMax
p_Session.setTrialMax(tmax)
Write a new maximum trial number. This should be an integer.
setBlockMax
p_Session.setBlockMax(bmax)
Write a new maximum block number. This should be an integer.
startTrial
p_Session.startTrial()
Begin the next trial in the session.
startBlock
p_Session.startBlock()
Begin the next block in the session.
endTrial
p_Session.endTrial()
End the current trial in the session.
Status
getTrialMax
p_Session.getTrialMax()
Read the maximum trial number (integer).
getBlockMax
p_Session.getBlockMax()
Read the maximum block number (integer).
curTrial
p_Session.curTrial()
Read the current trial number (integer).
curBlock
p_Session.curBlock()
Read the current block number (integer).
curSession
p_Session.curSession()
Read the current session number (integer).
isBlockStarting
p_Session.isBlockStarting()
See Flow Control to see when this returns true during the session.
isBlockEnding
p_Session.isBlockEnding()
See Flow Control to see when this returns true during the session.
isBlockNew
p_Session.isBlockNew()
See Flow Control to see when this returns true during the session.
isSessionStarting
p_Session.isSessionStarting()
See Flow Control to see when this returns true during the session.
isSessionEnding
p_Session.isSessionEnding()
See Flow Control to see when this returns true during the session.
isSessionActive
p_Session.isSessionActive()
See Flow Control to see when this returns true during the session.
isBlockActive
p_Session.isBlockActive()
See Flow Control to see when this returns true during the session.
isTrialActive
p_Session.isTrialActive()
See Flow Control to see when this returns true during the session.
Timers
markTime
p_Session.markTime(idx=1)
Start a custom timer (up to four can be used).
sinceRecordStart
Read the elapsed time since the recording began.
ts = p_Session.sinceRecordStart()
sinceSessionStart
Read the elapsed time since the current session began.
ts = p_Session.sinceSessionStart()
sinceBlockStart
Read the elapsed time since the current block began.
ts = p_Session.sinceBlockStart()
sinceTrialStart
Read the elapsed time since the current trial began.
ts = p_Session.sinceTrialStart()
sinceTrialEnd
Read the elapsed time since the last trial ended.
ts = p_Session.sinceTrialEnd()
sinceMark
ts = p_Session.sinceMark(idx=1)
Read a custom timer that was started with markTime
.