Tips and Tricks
Timeout Errors
If any method takes longer than ~3 seconds to execute, you will see this message in the Console Output window:
Timeout error while waiting for response from Python kernel
Avoid doing anything computationally intensive that takes longer than 3 seconds. Also, avoid
using time.sleep
statements as a way to control experiment flow. Use additional states
and setTimeout
to manage experiment flow.
Here's an example of poor design that will cause a timeout error:
import time
class State1: #StateID = ?
def s_Input1_rise():
print('button pressed')
time.sleep(5) # this will cause Timeout error
print('do something')
Instead, use the Pynapse state machine to keep track of the time delay for you:
class State1: #StateID = ?
def s_Input1_rise():
print('button pressed')
p_State.setTimeout(5, State2)
class State2:
def s_State_enter():
print('do something')
Synchronizing Events
By default, all outputs in the Python code are executed sequentially as they are
written. In the example below, outputs and timers are turned on in a slot method.
The time.sleep
statements are used for demonstration purposes to exaggerate the
effect by adding additional latency between each call.
import time
class MyState1: #StateID = 1
def s_MyInput_rise():
p_Output.MyOutput1.fire()
time.sleep(0.1)
p_Timer.MyTimer1.setPeriod(.1)
p_Timer.MyTimer1.setRepeats(3)
p_Timer.MyTimer1.start()
time.sleep(0.1)
p_Output.MyOutput2.fire()
time.sleep(0.1)
p_State.switch(MyState2)
The two outputs and the timer have 'Epoc Save' turned on. The runtime output looks like this:
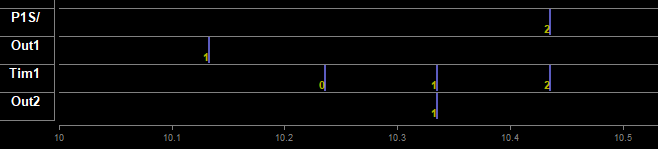
You can see the noticeable 100 ms gaps in between the output events, and all of these events occur before the state change ('P1S/' = 2 in this example).
For coordinating stimulus events or anything else that has to happen on the hardware simultaneously, the Outputs, Parameters, and Timers have a Sync to State Change option. If all of the outputs and timers in the last example had this option enabled, then the result looks like this:

The sleep
delays are still there but now all outputs fire precisely when the state
changed to 2.
Important
The p_State.switch
statement must come after any calls to set the timers or
outputs for this to work properly.
Delays
The polling loop delay depends on the 'Polling Rate' setting in the Pynapse General Tab. The typical round-trip delays (read Pynapse input → set Pynapse output) are shown below.
For tighter behavioral state control, always enable Maximum Polling Rate.
Note
Maximum polling rate is not available when using Corpus hardware emulation
Note
If using the SynapseAPI class in Pynapse, there is a variable delay that ranges from 5 to 30 ms. If the computer is under heavy processing, there can be delay spikes up to ~100 ms.
Important
Metric and Control asset 'writes' go through the SynapseAPI and have a longer delay.
Any calls that 'read' an asset value (except for Metric which are python variables) also go through the SynapseAPI.
Run-time Plots
If you would like to do online plotting or make your own custom GUIs then matplotlib and ipykernel==4.10.1 must also be installed in your Python environment.
If you want to plot something on screen using matplotlib you must include this line of code at the top of your Python code:
%matplotlib
Note about using a different matplotlib backend
If for some reason you need to set the matplotlib backend is set, once it is set
it cannot be changed for the entire interpreter session. For example, if in between
recordings you change %matplotlib qt
to %matplotlib tk
, the second statement
is ignored and qt backend will be used. If Pynapse code gets modified such that
a different backend is used, a complete restart of Synapse is required.