iCon Outputs
When an iCon is attached to the Pynapse Behavioral Controller in the General Tab, an additional iCon tab appears. This gives you a unified interface that lets you configure the iCon inputs/outputs directly within Pynapse and integrates them in the Python code editor.
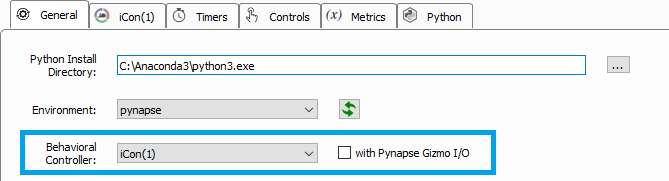
iCon Tab
Configure the iCon inputs/outputs in the iCon tab. See Logic Output Processor in the Synapse Manual for information on setting up the iCon outputs how to convert Pynapse function calls to physical hardware events.
![]() |
iCon Tab |
Run-time Interface
The run-time interface has a button for each output and an LED indicator that shows the current state of the output. For the iMn and iS9, additional options that were available during design-time can also be modified during run-time.
![]() |
iCon Run-time Interface |
Click an output to manually toggle its state (on/off). Hold down CTRL and click an output to 'mute' it. This prevents Pynapse from triggering the output.
Output Methods
All output methods have the form p_Rig.{OUTPUT_NAME}.{METHOD}
. Type p_
in the
Pynapse Code Editor and let the code completion do the work for you. The name of each
method gets replaced with the name of your actual output, so if you name the output
'Reward' then p_Rig.Reward.fire()
is an available method.
Manual Control
Manual turn outputs on, off, or fires a pulse waveform during runtime. Useful for stimulus/ reward presentation.
fire
p_Rig.MyOutput.fire()
This is only available if Triggered Pulse is selected.
Quickly pulse the output. If Duration is non-zero, the output will stay high for that set duration. Set Duration to zero to trigger a single sample pulse.
Example
Trigger an output when the input goes high.
class Always: #StateID = 0
def s_MyInput_pass():
p_Rig.MyOutput.fire()
turnOn
p_Rig.MyOutput.turnOn()
Turn the output on indefinitely. This is only available if Triggered Pulse is not selected.
Example
Link an input status to an output.
class Always: #StateID = 0
def s_MyInput_rise():
p_Rig.MyOutput.turnOn()
def s_MyInput_fall():
p_Rig.MyOutput.turnOff()
turnOff
p_Rig.MyOutput.turnOff()
Turn the output off. This is only available if Triggered Pulse is not selected.
Example
Link an input status to an output.
class Always: #StateID = 0
def s_MyInput_rise():
p_Rig.MyOutput.turnOn()
def s_MyInput_fall():
p_Rig.MyOutput.turnOff()
setMute
p_Rig.MyOutput.setMute(muted)
Mute the output so it can't trigger, or unmute it.
Duration Settings
setDuration
p_Rig.MyOutput.setDuration(dur_sec)
Override the output Duration setting. This is only available if Triggered Pulse is enabled and Duration is greater than 0.
Example
Modify the pulse shape and output value based on performance.
def s_State_enter():
# if more than 5 successful trials, decrease the output pulse time by 50 ms.
if p_Metric.success.read() > 5:
p_Metric.pulse_dur.dec(delta=0.05)
p_Rig.MyOutput.setDuration(p_Metric.pulse_dur.read())
Status
Get information on the current state of the output.
isOn
p_Rig.MyOutput.isOn()
Returns true if the output is currently true.
Example
When entering a state, check if an output is already true.
def s_state_enter():
if p_Rig.MyOutput.isOn():
print('MyOutput is on')
else:
print('MyOutput is off')
isOff
p_Rig.MyOutput.isOff()
Returns true if the output is currently false.
Example
When entering a state, check the status of the output.
def s_state_enter():
if p_Rig.MyOutput.isOff():
print('MyOutput is off')
else:
print('MyOutput is on')
iMn Output Settings
The iMn has analog outputs. These functions override the analog output settings at runtime. See iMn Analog Outputs for more information.
setAtten
p_Rig.MyOutput.setAtten(v)
Set the output Attenuation, in dB. This is only available if Waveform Shape is User, Tone, White Noise, Pink Noise, Square, Clock, or PWM.
setFreq
p_Rig.MyOutput.setFreq(v)
Set the output Frequency, in Hertz. This is only available if Waveform Shape is Tone, Square, or Clock.
setVolt
p_Rig.MyOutput.setVolt(v)
Set the output voltage, in Volts. This is only available if Waveform Shape is DC Voltage.
setDutyCycle
p_Rig.MyOutput.setDutyCycle(v)
Set the duty cycle percentage, from 0 to 100. This is only available if Waveform Shape is PWM.
Deprecated after v96
setPeriod
p_Rig.MyOutput.setPeriod(v)
Set the output period, in ms. This is only available if Waveform Shape is PWM.
setWidth
p_Rig.MyOutput.setWidth(v)
Set the output width, in ms. This is only available if Waveform Shape is PWM.
iS9 Output Settings
The iS9 sends a stimulation current. These functions override the output settings at runtime. See iS9 Stim Outputs for more information.
setStimCurrent
p_Rig.MyOutput.setStimCurrent(v)
Set the output current, in mA. This value ranges from 0.1 to 2.5 mA.